Shift register and LED:
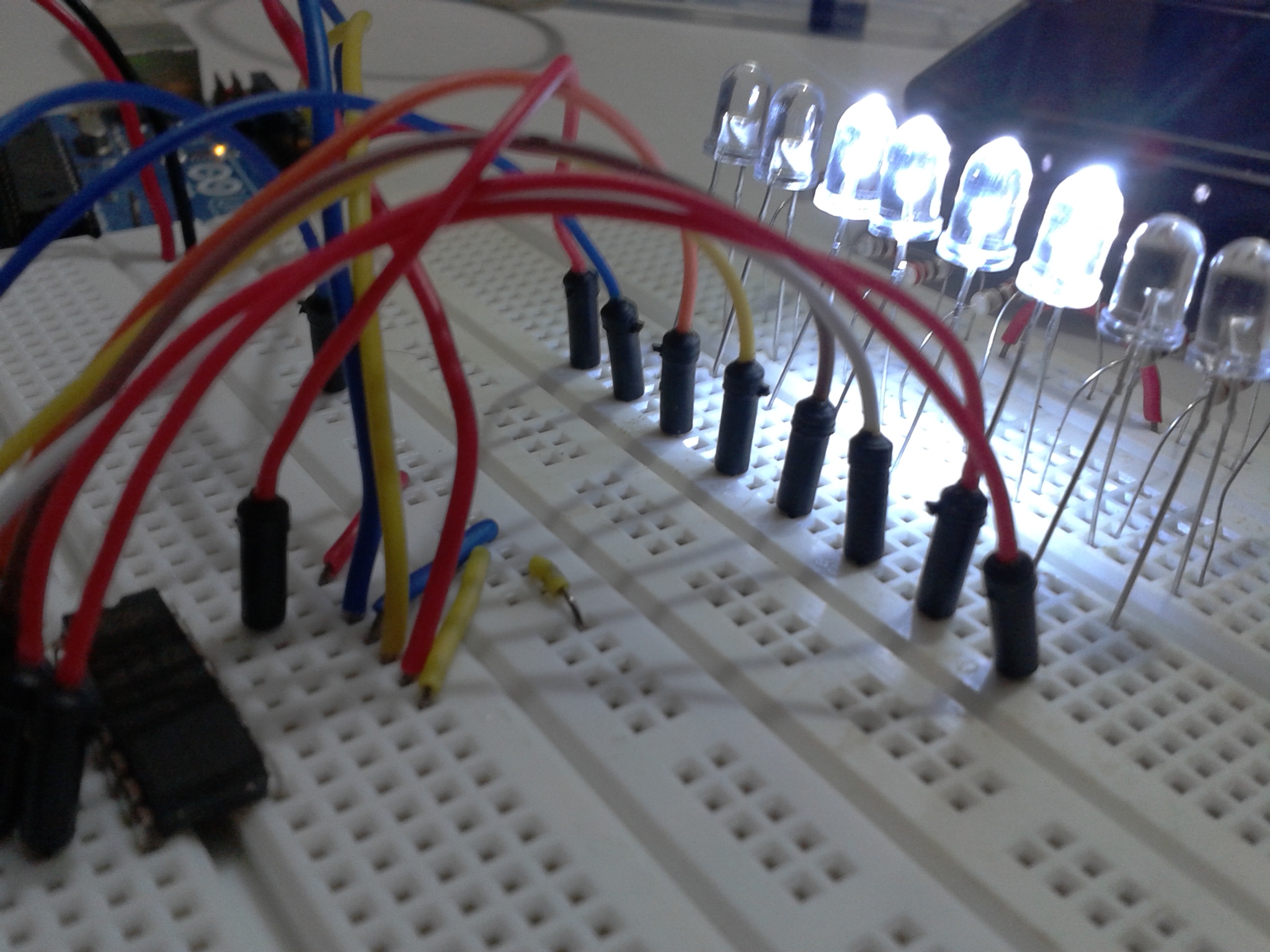
(for basic information on Shift Register, head over to Technopedia section)
Things needed:-
1) Arduino(any model will do)
2) 8 x LEDs
3) 1 x 74HC595N Shift register
Pin arrangement of the shift register is given in the following diagram:-
Connections:
Connect the circuit as per the schematic given below.

Code:-
Binary Counting
//visit http://inlovetech.ucoz.com for more projects
int dataPin = 2; //Define which pins will be used for the Shift Register control
int latchPin = 3;
int clockPin = 4;
int counter = 0; //The counter for storing the byte value
void setup()
{
pinMode(dataPin, OUTPUT); //Configure each IO Pin
pinMode(latchPin, OUTPUT);
pinMode(clockPin, OUTPUT);
}
void loop()
{
for (counter = 0; counter < 256; counter++)
{
digitalWrite(latchPin, LOW); //Pull latch LOW to start sending data
shiftOut(dataPin, clockPin, MSBFIRST, counter); //Send the data
digitalWrite(latchPin, HIGH); //Pull latch HIGH to stop sending data
delay(500);
}
}
LED Rider:-
//visit http://inlovetech.ucoz.com for more projects.
int dataPin = 2; //Define which pins will be used for the Shift Register control
int latchPin = 3;
int clockPin = 4;
int seq[14] = {1,2,4,8,16,32,64,128,64,32,16,8,4,2}; //The byte sequence
void setup()
{
pinMode(dataPin, OUTPUT); //Configure each IO Pin
pinMode(latchPin, OUTPUT);
pinMode(clockPin, OUTPUT);
}
void loop()
{
for (int n = 0; n < 14; n++)
{
digitalWrite(latchPin, LOW); //Pull latch LOW to start sending data
shiftOut(dataPin, clockPin, MSBFIRST, seq[n]); //Send the data
digitalWrite(latchPin, HIGH); //Pull latch HIGH to stop sending data
delay(75);
}
}
And thats it !!! JUST UPLOAD THE CODE AND HAVE FUN !!!